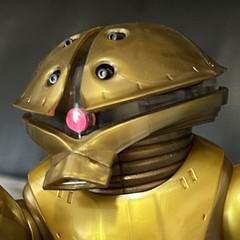
By Dark PhaZe Go To PostWhat are some of the first tasks you guys were given in your first few jobs in the industry?Learn how to create a website using java faclets (I think that's what it was called, it was a decade ago).
Learn the structure of the medical record database and create reports from it.
Learn how to create a responsive web app.
Design the framework for a MVC and Entity Framework-based website.
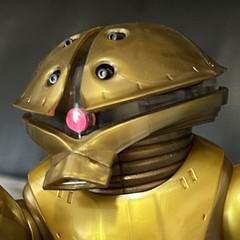
I need some help with React + material-ui. I am trying to create a SearchBox component where both typing in the box and clicking on a SearchIcon within the SearchBox fire an onChange event that can be handled by the parent component. I am struggling to even figure out how to get to the parent TextField element from within the IconButton element in a React kind of way.


import React from "react"
import IconButton from "@material-ui/core/IconButton";
import InputAdornment from "@material-ui/core/InputAdornment";
import SearchIcon from '@material-ui/icons/Search';
import TextField from '@material-ui/core/TextField'
const SearchBox = ({label, onChange}) => {
const handleClick = (event) => {
// TODO: fire TextField's onChange event
}
return <TextField label={label} onChange={onChange} variant="filled" InputProps={{
endAdornment: (
<InputAdornment>
<IconButton onClick={handleClick}>
<SearchIcon/>
</IconButton>
</InputAdornment>)
}}/>
};
export default SearchBox
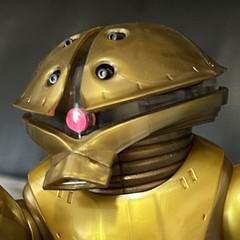
Hmmm... after thinking on it for a bit, should I create a State variable in the TextField tied to the value and have it updated on the TextField onChange event and have a separate onIconClick event passed in that can return the value of TextField using that State variable?
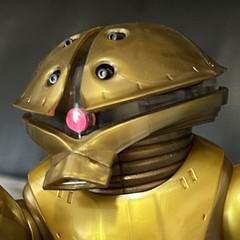
Ah hah! It worked!
e: refactored it a little bit
import React, {useState} from "react"
import IconButton from "@material-ui/core/IconButton";
import InputAdornment from "@material-ui/core/InputAdornment";
import TextField from '@material-ui/core/TextField'
import SearchIcon from '@material-ui/icons/Search';
const SearchBox = ({label, onChange, onIconClick}) => {
const [searchText, setSearchText] = useState('');
const handleChange = (event) => {
const eventTargetValue = event.target.value;
setSearchText(eventTargetValue);
onChange(event, eventTargetValue);
}
const handleIconClick = (event) => {
onIconClick(event, searchText);
}
return <TextField label={label} onChange={handleChange} variant="filled" InputProps={{
endAdornment: (
<InputAdornment>
<IconButton onClick={handleIconClick}>
<SearchIcon/>
</IconButton>
</InputAdornment>)
}}/>
};
export default SearchBox
e: refactored it a little bit
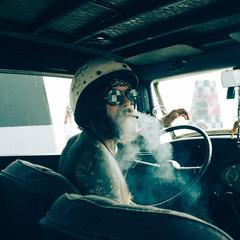
That's correct. useState is the way to go.
Let me know how hooks are, React is going fully functional rather than class lifecycle based which is exciting.
Your next step is to learn Redux as a state management framework and using it to integrate with an API. After redux you should learn Redux selectors to learn how to derive computed data. Then Redux reselect to learn how to memoize your selectors.
Let me know how hooks are, React is going fully functional rather than class lifecycle based which is exciting.
Your next step is to learn Redux as a state management framework and using it to integrate with an API. After redux you should learn Redux selectors to learn how to derive computed data. Then Redux reselect to learn how to memoize your selectors.
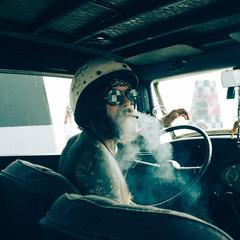
To be specific, there's two types of state you need to worry about:
- Localized component state utilized with hooks or a class state. This is for storing component specific temporary data eg the value of a search form before you submit like you are doing above.
- Global state for the entire app using something like Redux, Flux, Nuclear.js, etc is meant to be global state that you ingest your API data with and interact with as a shared state for the entire application.
The other thing you should eventually learn is higher-order-components, a nice composition pattern that lets you have shared logic at a parent component that your child component shares.
- Localized component state utilized with hooks or a class state. This is for storing component specific temporary data eg the value of a search form before you submit like you are doing above.
- Global state for the entire app using something like Redux, Flux, Nuclear.js, etc is meant to be global state that you ingest your API data with and interact with as a shared state for the entire application.
The other thing you should eventually learn is higher-order-components, a nice composition pattern that lets you have shared logic at a parent component that your child component shares.
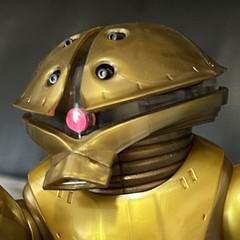
Aside from some other practice examples/tutorials, this was my first real experience with hooks. I mostly used react's own introduction to learn more about them: https://reactjs.org/docs/hooks-state.html
I did not know that react was moving to become functional throughout, but just from learning it from scratch and with my previous javascript experience, it feels more natural for it to just be functional everywhere. The classes honestly felt out of place.
It might be a little while before I get into learning Redux because the next step in this personal project is to query from a Firebase instance. The tutorials that I have been reading on it have been using HOCs to handle connecting to the instance, querying it, and managing sessions so I understand them on a surface level. Will have to play around with it a bit before I really understand and feel comfortable with it and how it works.
I did not know that react was moving to become functional throughout, but just from learning it from scratch and with my previous javascript experience, it feels more natural for it to just be functional everywhere. The classes honestly felt out of place.
It might be a little while before I get into learning Redux because the next step in this personal project is to query from a Firebase instance. The tutorials that I have been reading on it have been using HOCs to handle connecting to the instance, querying it, and managing sessions so I understand them on a surface level. Will have to play around with it a bit before I really understand and feel comfortable with it and how it works.
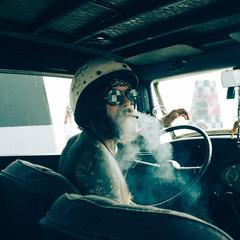
To be clear, they won't get rid of classes: https://reactjs.org/docs/hooks-intro.html and https://reactjs.org/docs/hooks-faq.html#should-i-use-hooks-classes-or-a-mix-of-both
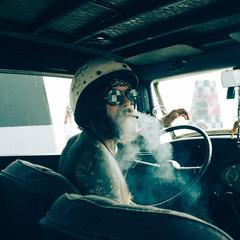
This is a good guide when it comes to Redux and Hooks https://react-redux.js.org/api/hooks
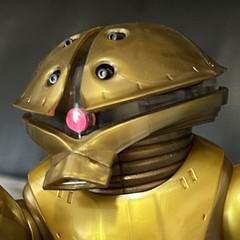
Spent most of this week and a good portion of last week doing some React work with Gatsby and a CMS system for work and I have to say that developing in this kind of framework is really just interesting and enjoyable. I like it.
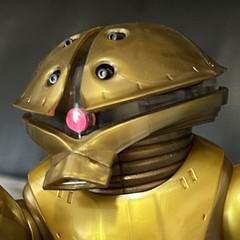
Learned how to write and work with GraphQL fragments this past weekend because I had an object with a bunch of images and I wanted them all to be formatted the same as each other so I created a fragment for that. I also figured out how to move a GraphQL query out to it's own file so that it's hugeness doesn't bloat my component's main code file.
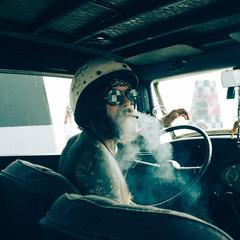
Let me know how your GraphQL interaction is. Mine was extremely unpleasant but that might have been that I was working with somebody else's incredibly bad implementation of it.
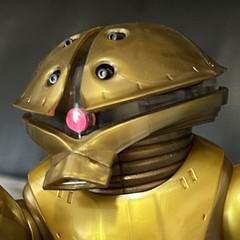
By reilo Go To PostLet me know how your GraphQL interaction is. Mine was extremely unpleasant but that might have been that I was working with somebody else's incredibly bad implementation of it.It's been fairly nice, tbh. The CMS vendor I am using showed me how to use a resolver in order to get a collection inside of a collection and how to use the remoteFileNode function in Gatsby to pull down images stored in the CMS system to my local system for processing.
The only thing that has been kind of weird is the Gatsby optimized image thing. You can't use the fragment
...GatsbyImageSharpFluid
in the GraphQL designer (it throws an error) but still need to use it in the query in your code base.Once I got the collection inside a collection and remote images stuff working, that fragment for optimized images is the only complaint I really have with it.
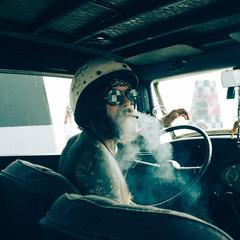
My primary issue is that it's not well suited for a mobile app since it requires a generated schema to be available to do the type validation. So, for example, the way it was doing the type validation was extremely strict so when a new variant of something was introduced it required an app store release, which isn't very sustainable especially if you have a product catalog that need to change on the fly. We had several issues where we couldn't release something new without things breaking, and that was just mind-boggling.
The other was that it was a very new framework and concept so it was prone to all of those pitfalls that came with it. Pretty sure halfway into development they released a new GraphQL spec standard that was incompatible with their original.
In theory it should make things easier and provide flexibility for developers, but the entire concept is all-in or nothing and it's very opinionated.
The other was that it was a very new framework and concept so it was prone to all of those pitfalls that came with it. Pretty sure halfway into development they released a new GraphQL spec standard that was incompatible with their original.
In theory it should make things easier and provide flexibility for developers, but the entire concept is all-in or nothing and it's very opinionated.
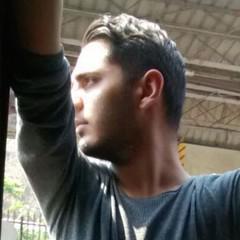
By Kibner Go To PostAh hah! It worked!Using state is definitely a solid way of doing that, but you may also want to look at `useRef` in the case where you want to do a bit more with the input and don't want to actively update the state on every change.import React, {useState} from "react" import IconButton from "@material-ui/core/IconButton"; import InputAdornment from "@material-ui/core/InputAdornment"; import TextField from '@material-ui/core/TextField' import SearchIcon from '@material-ui/icons/Search'; const SearchBox = ({label, onChange, onIconClick}) => { const [searchText, setSearchText] = useState(''); const handleChange = (event) => { const eventTargetValue = event.target.value; setSearchText(eventTargetValue); onChange(event, eventTargetValue); } const handleIconClick = (event) => { onIconClick(event, searchText); } return <TextField label={label} onChange={handleChange} variant="filled" InputProps={{ endAdornment: ( <InputAdornment> <IconButton onClick={handleIconClick}> <SearchIcon/> </IconButton> </InputAdornment>) }}/> }; export default SearchBox
e: refactored it a little bit
https://reactjs.org/docs/hooks-reference.html#useref
Also, in the case where you're storing the value in state, you may as well also turn your TextField into a controlled component (provide that value back to the component)
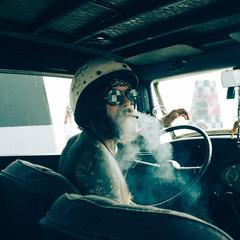
I've personally always considered uncontrolled inputs an anti-pattern in React. There's valid reasons for doing it that way, but they are super super rare. I'd highly suggest keeping it a controlled component.
A good primer on both: https://goshakkk.name/controlled-vs-uncontrolled-inputs-react/
A good primer on both: https://goshakkk.name/controlled-vs-uncontrolled-inputs-react/
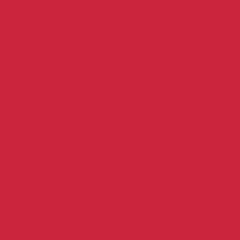
Completely green when it comes to programming. What are the best resources that can help me step-by-step in learning Python? I’ve downloaded Pythonista on my phone and did some addition, multiplication. Then I got stuck on user inputs and figure I need some for dummies tutorial. Anyone have suggestions?
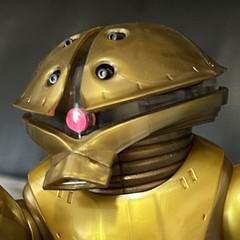
By Blue Go To PostCompletely green when it comes to programming. What are the best resources that can help me step-by-step in learning Python? I’ve downloaded Pythonista on my phone and did some addition, multiplication. Then I got stuck on user inputs and figure I need some for dummies tutorial. Anyone have suggestions?Dang, you just barely missed the boat to apply for a free Standford course teaching Python. I don't know any good tutorials off-hand, but the official site has one: https://docs.python.org/3/tutorial/index.html
I imagine it should be pretty good about teaching best practices for the simple things.
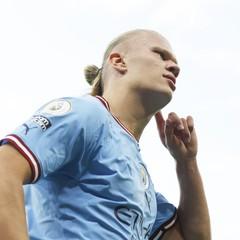
I always see people recommend Learn Python the Hard Way, and datacamp has some nice tutorials - you can get two months free through Visual Studio Dev Essentials
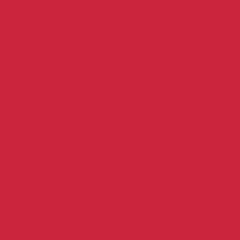
Just got this off Udemy for free: https://www.udemy.com/course/automate/?couponCode=MAY2020FREE2
Gonna load up on YouTube videos as well. Thanks for suggestions.
Gonna load up on YouTube videos as well. Thanks for suggestions.
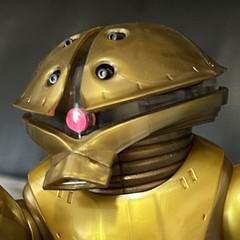
My gf has been doing that Stanford Python programming course for three weeks now. It is her first time learning programming, though she did know if/then statements and while loops from playing EXAPUNKS. Anyway, here is what she made yesterday, entirely in Python:

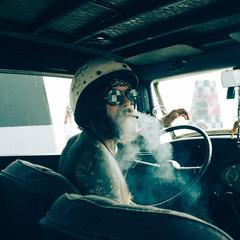
Microsoft has admitted it was wrong about open source, after the company battled it and Linux for years at the height of its desktop domination. Former Microsoft CEO Steve Ballmer famously branded Linux “a cancer that attaches itself in an intellectual property sense to everything it touches” back in 2001.https://www.theverge.com/2020/5/18/21262103/microsoft-open-source-linux-history-wrong-statement
Microsoft president Brad Smith now believes the company was wrong about open source. “Microsoft was on the wrong side of history when open source exploded at the beginning of the century, and I can say that about me personally,” said Smith in a recent MIT event. Smith has been at Microsoft for more than 25 years and was one of the company’s senior lawyers during its battles with open-source software.
“The good news is that, if life is long enough, you can learn … that you need to change,” added Smith. Microsoft has certainly changed since the days of branding Linux a cancer. The software giant is now the single largest contributor to open-source projects in the world, beating Facebook, Docker, Google, Apache, and many others.
Good Guys Microsoft™
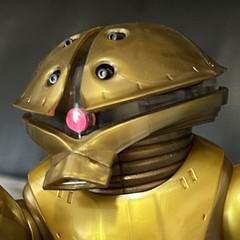
My gf made this in about a week for her final project for that Standard Code in Place course (which was about five weeks and was her first exposure to programming):
She made it using PyGame (and other assorted libraries). Most of the sprites were ripped from a Zelda game but she had to clean them up and adjust their animations, anyway.
That little robot thing is what the students had to use in most of their first programs. It is called Karel and it had to place and pickup beepers in their earlier assignments. But it didn't have any animations or anything really. Just moved instantly from one tile to another so she had to do a good bit of work to actually animate them and have them moving.
She learned so much and I'm so damn proud of her.
She made it using PyGame (and other assorted libraries). Most of the sprites were ripped from a Zelda game but she had to clean them up and adjust their animations, anyway.
That little robot thing is what the students had to use in most of their first programs. It is called Karel and it had to place and pickup beepers in their earlier assignments. But it didn't have any animations or anything really. Just moved instantly from one tile to another so she had to do a good bit of work to actually animate them and have them moving.
She learned so much and I'm so damn proud of her.
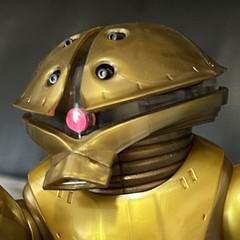
Oh, she also said that this youtube channel helped her a lot when making that simple game: https://www.youtube.com/channel/UCNaPQ5uLX5iIEHUCLmfAgKg. It's called "Kids Can Code" and she said the guy gave very good explanations and examples on how to do things.
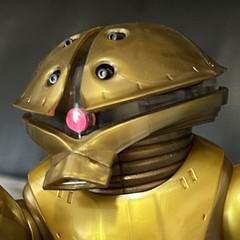
I learned a lot about IPv6 in the last week. :v I posted in the PC Hardware thread about getting a Pi-hole setup in Docker and my struggles with IPv6 and how I eventually got it working. Since then, I have learned more about IPv6 and Docker and now have it working properly. Below is a revised guide of what I had to do.
First thing I did was find the IP my router/gateway was on and used the Global ID defined in it as the basis for the rest of my user-defined IPv6 network. Then, I edited /etc/network/interfaces to set a static IPv6 for my host machine since my router is unable to do that. The X's are placeholders for the global ID of my IPv6 network.
/etc/network/interfaces
The next step was to enable IPv6 in Docker. By default, Docker has this disabled. Docker also does not automatically assign/allocate IPv6 addresses, so we have to do this manually ourselves. The "fixed-cidr-v6" variable is for the default Docker bridge network. I have it using a different subnet from my host server and router just because I want to. I do not know if it actually brings better security or isolation or anything.
/etc/docker/daemon.json
I then rebooted my machine at this point to get all the services to reset. If I was smarter and more familiar with Linux/Debian, I would have just restarted only the necessary services instead of the whole machine. As this is a home server that isn't running anything vital, I didn't bother.
Next, I created an IPv6 network for the Pi-hole. Very similar to what I did for the default Docker bridge network, I assigned a unique subnet to the existing Global ID for my user-defined IPv6 network. I created and saved a small script for this:
docker-network_create.sh
Ran the script and verified that it was successfully created.
Next, I created a file for docker-compose to run. The key thing I had to do here was set ServerIPv6 to the static IP of my host server that I set in the beginning in the /etc/network/interfaces file.
docker-compose.yaml
Ran
First thing I did was find the IP my router/gateway was on and used the Global ID defined in it as the basis for the rest of my user-defined IPv6 network. Then, I edited /etc/network/interfaces to set a static IPv6 for my host machine since my router is unable to do that. The X's are placeholders for the global ID of my IPv6 network.
/etc/network/interfaces
[...]
iface eno1 inet6 static
address fdXX:XXXX:XXXX:1:0:0:0:25
netmask 64
gateway fdXX:XXXX:XXXX:1:0:0:0:1
The next step was to enable IPv6 in Docker. By default, Docker has this disabled. Docker also does not automatically assign/allocate IPv6 addresses, so we have to do this manually ourselves. The "fixed-cidr-v6" variable is for the default Docker bridge network. I have it using a different subnet from my host server and router just because I want to. I do not know if it actually brings better security or isolation or anything.
/etc/docker/daemon.json
{
"ipv6": true,
"fixed-cidr-v6": "fdXX:XXXX:XXXX:b35d::/64"
}
I then rebooted my machine at this point to get all the services to reset. If I was smarter and more familiar with Linux/Debian, I would have just restarted only the necessary services instead of the whole machine. As this is a home server that isn't running anything vital, I didn't bother.
Next, I created an IPv6 network for the Pi-hole. Very similar to what I did for the default Docker bridge network, I assigned a unique subnet to the existing Global ID for my user-defined IPv6 network. I created and saved a small script for this:
docker-network_create.sh
docker network create --ipv6 --subnet "172.18.10.0/24" --subnet "fdXX:XXXX:XXXX:629f::/64" pi-hole
Ran the script and verified that it was successfully created.
Next, I created a file for docker-compose to run. The key thing I had to do here was set ServerIPv6 to the static IP of my host server that I set in the beginning in the /etc/network/interfaces file.
docker-compose.yaml
version: "3"
# More info at https://github.com/pi-hole/docker-pi-hole/ and https://docs.pi-hole.net/
services:
pihole:
container_name: pihole
image: pihole/pihole:latest
ports:
- "53:53/tcp"
- "53:53/udp"
- "80:80/tcp"
- "443:443/tcp"
environment:
TZ: 'America/Chicago'
WEBPASSWORD: somePassword
DNS1: 9.9.9.9
DNS2: 149.112.112.112
DNSSEC: "true"
CONDITIONAL_FORWARDING: "true"
CONDITIONAL_FORWARDING_IP: 192.168.7.1
CONDITIONAL_FORWARDING_REVERSE: 1.7.168.192.in-addr.arpa
ServerIP: 192.168.7.8
ServerIPv6: fdXX:XXXX:XXXX:1:0:0:0:25
# Volumes store your data between container upgrades
volumes:
- './docker-volumes/etc-pihole/:/etc/pihole/'
- './docker-volumes/etc-dnsmasq.d/:/etc/dnsmasq.d/'
dns:
- 127.0.0.1
- 9.9.9.9
restart: unless-stopped
networks:
default:
external:
name: pi-hole
Ran
docker-compose up -d
and that was it.
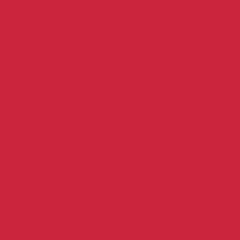
In python. When I define my function, does it matter if I place my variables/parameter-values before or after the function?
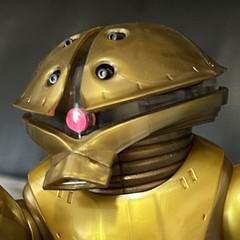
By Blue Go To PostIn python. When I define my function, does it matter if I place my variables/parameter-values before or after the function?I don't believe it matters but it is generally good practice to have all your file/class scoped variables at the top of the file.
Are you able to post some sample code for us to look at? I may be misunderstanding what you are asking.
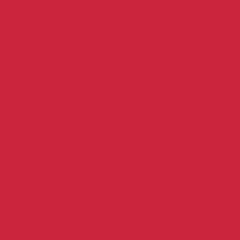
By Kibner Go To PostI don't believe it matters but it is generally good practice to have all your file/class scoped variables at the top of the file.
Are you able to post some sample code for us to look at? I may be misunderstanding what you are asking.
def isFirst_And_Last_Same(numberList):
print("Given list is ", numberList)
firstElement = numberList[0]
lastElement = numberList[-1]
if (firstElement == lastElement):
return True
else:
return False
numList = [10, 20, 30, 40, 10]
print("result is", isFirst_And_Last_Same(numList))
Can numList be before the function instead of after? Keep seeing it after on these Python exercises and wondering why it couldn’t be before the function.
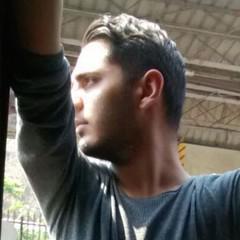
It _can_ be before or after, it's being passed into the function anyway, so it really doesn't matter, so long as it's defined before the function call
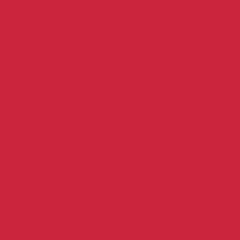
By JesalR Go To PostIt _can_ be before or after, it's being passed into the function anyway, so it really doesn't matter, so long as it's defined before the function callI figured. Helps me writing my function if those are defined before instead of after. Thanks.
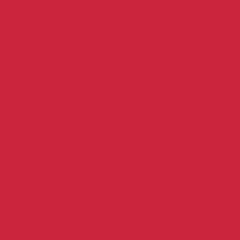
So strings are immutable in Python. So I want to understand this code:
task = “Subscribe”
print(id(task))
task = “Like”
print(id(task))
print(task)
Returns two different id’s and the string Like. If strings are immutable why can subscribe be changed to like and why are the id’s different? I’m sure there are good reasons but I can’t figure out why.
task = “Subscribe”
print(id(task))
task = “Like”
print(id(task))
print(task)
Returns two different id’s and the string Like. If strings are immutable why can subscribe be changed to like and why are the id’s different? I’m sure there are good reasons but I can’t figure out why.
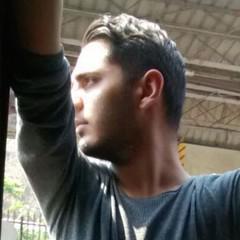
By Blue Go To PostSo strings are immutable in Python. So I want to understand this code:The string is immutable, but you're reassigning the variable to a new string. On the bolded line, the task string is pointing at one place in memory, in the underlined line, it's changed to point to somewhere else.
task = “Subscribe”
print(id(task))
task = “Like”
print(id(task))
print(task)
Returns two different id’s and the string Like. If strings are immutable why can subscribe be changed to like and why are the id’s different? I’m sure there are good reasons but I can’t figure out why.
That's why id returns a different value each time, because each is a different object.
The variable is mutable, the value is not
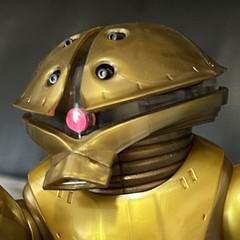
I don't really know python but I assume the interpreter creates a new string instance when you do that. This quora link seems to back me up: https://www.quora.com/What-does-immutable-mean-Which-data-types-in-Python-are-immutable
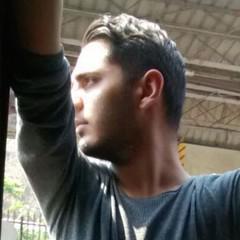
Another way of looking at it is
X is created as a reference to the string world. Y is set to that same reference, the reference of X is then changed to a new string object hello
x = "world"
y = x
print(x, y) # prints "world world"
x = "hello"
print(x, y) # prints "hello world"
X is created as a reference to the string world. Y is set to that same reference, the reference of X is then changed to a new string object hello
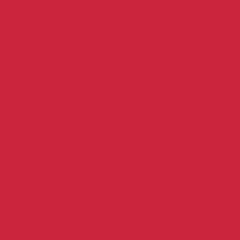
By JesalR Go To PostThe string is immutable, but you're reassigning the variable to a new string. On the bolded line, the task string is pointing at one place in memory, in the underlined line, it's changed to point to somewhere else.I see. I got fixated on strings being immutable that I didn’t realize I didn’t actually attempt any changes to the string. When you say “pointing at one place in memory” you mean RAM? I just ran the that code again and both ID’s change.
That's why id returns a different value each time, because each is a different object.
The variable is mutable, the value is not
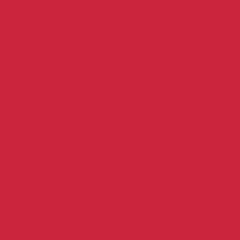
By Kibner Go To PostI don't really know python but I assume the interpreter creates a new string instance when you do that. This quora link seems to back me up: https://www.quora.com/What-does-immutable-mean-Which-data-types-in-Python-are-immutableVery helpful link. 👍
I agree with your interpretation.
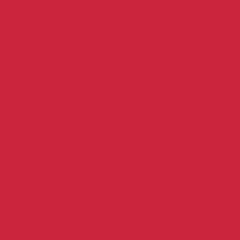
And thanks for the reply’s. I google on Reddit, Quora and Stackoverflow but sometimes their explanations are so confusing and hard to follow. Just give me the highlights for now. 😂
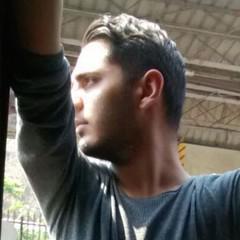
By Blue Go To PostI see. I got fixated on strings being immutable that I didn’t realize I didn’t actually attempt any changes to the string. When you say “pointing at one place in memory” you mean RAM? I just ran the that code again and both ID’s change.Yes, in ram, although it's not guaranteed that the place in memory will be different, but that's unimportant for now.
With strings in python and some other languages like js, anything that alters a string will actually return a new string
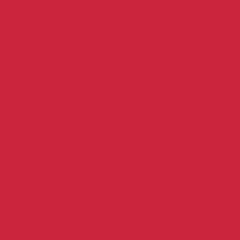
while True:
print("Continue? Y/N")
response = input()
if response.lower() != "y":
break
So is this the same thing as saying if response is not equal to "Y' or not equal to "y" break?
print("Continue? Y/N")
response = input()
if response.lower() != "y":
break
So is this the same thing as saying if response is not equal to "Y' or not equal to "y" break?
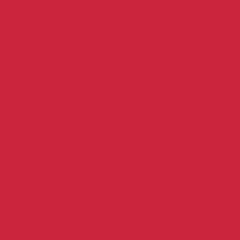
By JesalR Go To PostYes, that should be what happens thereIf I type “Y” as my input, “Y” and lower case “y” are being checked at the same time against the not equal “y”?
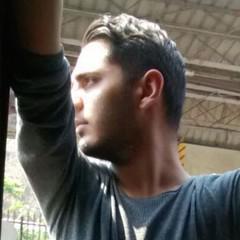
By Blue Go To PostIf I type “Y” as my input, “Y” and lower case “y” are being checked at the same time against the not equal “y”?Not quite. Whatever you type is being converted to a lowercase version, that lowercase version is then checked against "y"
Since not both versions are being compared (the original and lowercase), the following will always `break` regardless of what you enter
while True:
print("Continue? Y/N")
response = input()
if response.lower() != "Y":
break
If your response is "Y", it gets converted to "y" and "y" != "Y" so it will hit the break
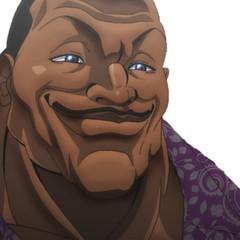
Got some Android programming experience recently. It's....ok? I can't say I love using emulators. Are you guys pretty experienced with making native mobile apps in general? Just for curiosities sake.
Completely unrelated--Windows is becoming pretty tiresome in general. Considering dual booting to Linux and creating separation between professional stuff and gaming.
Completely unrelated--Windows is becoming pretty tiresome in general. Considering dual booting to Linux and creating separation between professional stuff and gaming.