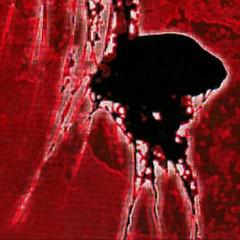
When this goes open source, I'm down to contribute. I'll brush up on my python/Django and PostgreSQL until then :).
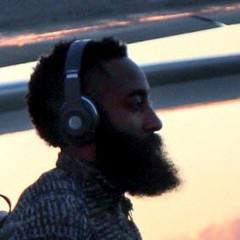
By reilo Go To PostFYI, if anyone is looking to contribute to SLAENT in any capacity from a development perspective, please feel free to hit me up. I already set up Kibner last weekend.I'm not a dev, but I'm professionally a sysadmin. Might be able to help with tasks related to hardware, networking or OS.
Basically, we run on Python 3.5 using Django, Postgres and a React front-end via ES2017 transpiled down via Babel.
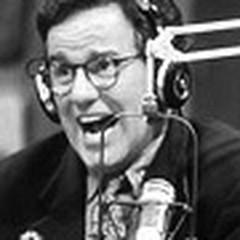
By c++ Go To PostLet's be honest, we all know there's only one true language.
Latin.
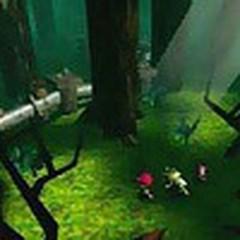
By Randolph Freelander Go To PostBy c++ Go To PostLet's be honest, we all know there's only one true language.Latin.
Veritas.
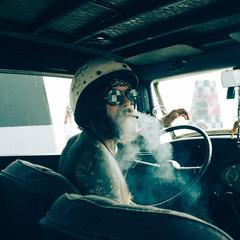
By c++ Go To PostLet's be honest, we all know there's only one true language.Lisp.
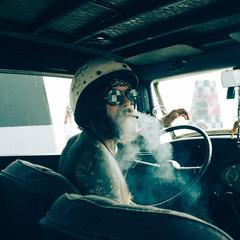
By vic Go To PostI'm not a dev, but I'm professionally a sysadmin. Might be able to help with tasks related to hardware, networking or OS.Word. Servers are seemingly in solid shape right now. I'll posit some Qs if I am stuck on something!
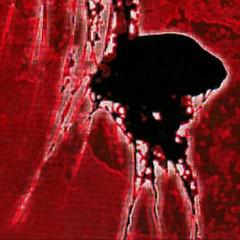
By c++ Go To PostLet's be honest, we all know there's only one true language.
C + lojure
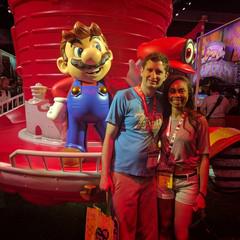
I'm a game developer so I've not done much with web programming or IT, but I'd be glad to try to pitch in where I can.
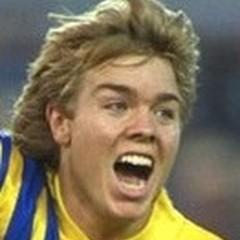
By c++ Go To PostLet's be honest, we all know there's only one true language.My man.
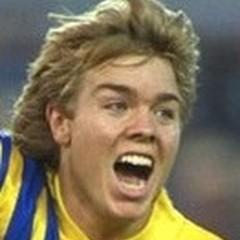
I was close to losing a day's worth of work today. Don't forget to push/commit/backup regularly lads.
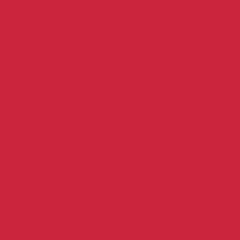
It’s always funny listening to people who are just starting to learn programming and what their adventures are. Especially when they land their first job as a junior developer for a large company and realize that most of the code is classic ASP or FoxPro with stored procedures controlling all of the business logic as well as having to integrate with an AS400 backend and deal with the one legacy RPG programmer that’s been with the company since the 80’s. REST? IoC? Containers? MVC? Lolwut?
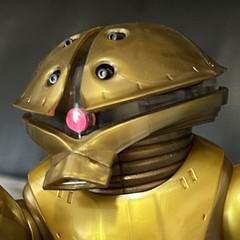
Please stop reminding me. I'm trying to move out of the bad times.
Though I am probably spending tomorrow coding up a grid for use in the admin configuration of a joomla module and that is almost as bad. You basically have to write all your javascript and html as a string and load it into the page via php.
Though I am probably spending tomorrow coding up a grid for use in the admin configuration of a joomla module and that is almost as bad. You basically have to write all your javascript and html as a string and load it into the page via php.
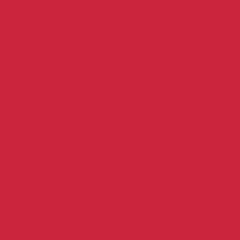
By Kibner Go To PostPlease stop reminding me. I'm trying to move out of the bad times.
Though I am probably spending tomorrow coding up a grid for use in the admin configuration of a joomla module and that is almost as bad. You basically have to write all your javascript and html as a string and load it into the page via php.
Sounds like a waste adding technical debt ;)
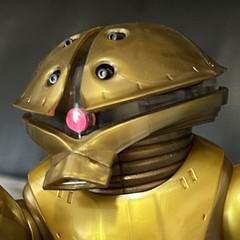
By eclipze Go To PostSounds like a waste adding technical debt ;)Yes, but it is a (dumb) requirement. *sigh*
e: thankfully, my ide can still correctly parse and apply syntax highlighting and all that goodness when it detects i'm putting html or js in a string so it's not as bad as it could be
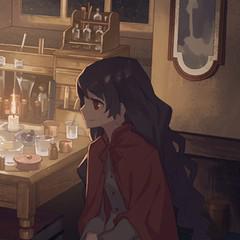
I need help with some C++ programming.
So my task is to convert from a given calendar date to an unsigned serial (which I could do) and then from the serial back to a calendar date (which I can't do).
The serial counts the number of days from 1-1-1900.
My function to convert a calendar date is this.
Contained within a Date class:
unsigned serial() const
{
return days_epoch[m_y - 1900] + day_of_year();
}
Contained within a cpp file
unsigned Date::day_of_year() const
{
return days_ytd[m_m - 1] + ((m_m > 2 && m_is_leap) ? 1 : 0) + (m_d - 1);
}
const std::array<unsigned, 12=""> Date::days_in_month = { {31,28,31,30,31,30,31,31,30,31,30,31} };
const std::array<unsigned, 12=""> Date::days_ytd{ {0,31,59,90,120,151,181,212,243,273,304,334} };
const std::array<unsigned, date::n_years=""> Date::days_epoch(static_cast<const std::array<unsigned,="" date::n_years="">&>(DateInitializer()));
DateInitializer()
{
for (unsigned j = 0, s = 0, y = Date::first_year; j < size(); ++j, ++y)
{
(*this)[ j ] = s;
s += 365 + (Date::is_leap_year(y) ? 1 : 0);
}
}
bool Date::is_leap_year(unsigned year)
{
return ((year % 4 != 0) ? false : (year % 100 != 0) ? true : (year % 400 != 0) ? false : true);
}
So this is how the code would work.
My question, once again, is, how do I create a function that prints the calendar date to a string from a given serial input?
Thanks
So my task is to convert from a given calendar date to an unsigned serial (which I could do) and then from the serial back to a calendar date (which I can't do).
The serial counts the number of days from 1-1-1900.
My function to convert a calendar date is this.
Contained within a Date class:
unsigned serial() const
{
return days_epoch[m_y - 1900] + day_of_year();
}
Contained within a cpp file
unsigned Date::day_of_year() const
{
return days_ytd[m_m - 1] + ((m_m > 2 && m_is_leap) ? 1 : 0) + (m_d - 1);
}
const std::array<unsigned, 12=""> Date::days_in_month = { {31,28,31,30,31,30,31,31,30,31,30,31} };
const std::array<unsigned, 12=""> Date::days_ytd{ {0,31,59,90,120,151,181,212,243,273,304,334} };
const std::array<unsigned, date::n_years=""> Date::days_epoch(static_cast<const std::array<unsigned,="" date::n_years="">&>(DateInitializer()));
DateInitializer()
{
for (unsigned j = 0, s = 0, y = Date::first_year; j < size(); ++j, ++y)
{
(*this)[ j ] = s;
s += 365 + (Date::is_leap_year(y) ? 1 : 0);
}
}
bool Date::is_leap_year(unsigned year)
{
return ((year % 4 != 0) ? false : (year % 100 != 0) ? true : (year % 400 != 0) ? false : true);
}
So this is how the code would work.
My question, once again, is, how do I create a function that prints the calendar date to a string from a given serial input?
Thanks
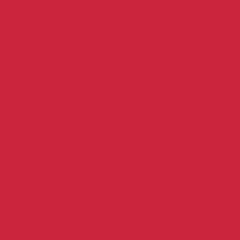
I haven't finished filling out the details yet because I have to run to lunch, but I started from scratch and came up with something like this. I need to fill out a few more methods, and I haven't even compiled this so there could be issues. Will finish up later.
Edit: Ok fixed it up. Again, I haven't even compiled this, and this seemed way more complicated than I expected it to be, so maybe there's an easier solution.
const unsigned days_per_month[] = {
31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31
};
constexpr unsigned seconds_per_day = 24 * 60 * 60;
constexpr unsigned seconds_per_nl_year = 365 * seconds_per_day;
constexpr unsigned seconds_per_l_year = 366 * seconds_per_day;
// Number of seconds per century where the centennial is not a
// leap year.
constexpr unsigned leaps_per_nl_century = 24;
constexpr unsigned seconds_per_nl_century
= leaps_per_nl_century * seconds_per_l_year
+ (100 - leaps_per_nl_century) * seconds_per_nl_year;
// Number of seconds per century where the centennial is a
// leap year.
constexpr unsigned leaps_per_l_century = 25;
constexpr unsigned seconds_per_l_century
= leaps_per_l_century * seconds_per_l_year
+ (100 - leaps_per_l_century) * seconds_per_nl_year;
// Number of seconds in a 4 year period that does include
// a leap year.
constexpr unsigned seconds_per_l_4_years =
3 * seconds_per_nl_year + seconds_per_l_year;
// centennial period is 400 years. For example
// 1900 - non-leap centennial
// 2000 - leap centennial
// 2100 - non-leap centennial
// 2200 - non-leap centennial
// at which point this repeats.
constexpr unsigned seconds_per_centennial_period
= 3 * seconds_per_nl_century
+ seconds_per_l_century;
class Date {
private:
unsigned year; // assert(year >= 1900)
unsigned month; // month in [0,12)
unsigned day; // day in [0,31)
// Given a number of seconds from Jan 1 for a particular
// year, find the corresponding month and day in that year.
static void which_month_and_day(unsigned seconds, unsigned year,
unsigned &m, unsigned &d) {
bool leap = is_leap_year(year);
for (int i=0; i < 12; ++i) {
unsigned dpm = days_per_month(i);
unsigned seconds_this_month = dpm * seconds_per_day;
if (leap && (i == 1))
seconds_this_month += seconds_per_day;
if (seconds < seconds_this_month) {
m = i;
d = seconds_this_month / seconds_per_day;
return;
}
seconds -= spm;
}
assert(false && "Invalid seconds count!");
}
// Given the number of seconds into a centennial period, which
// century are we in? On output, returns the index of the century
// we're in within this period, and sets "seconds" to contain the
// number of seconds into this century that we are.
static unsigned which_century_in_period(unsigned &seconds) {
assert(seconds < seconds_per_centennial_period);
unsigned s[] = {seconds_per_nl_century, seconds_per_l_century};
unsigned indices[] = {0, 1, 0, 0};
for (unsigned i=0; i < 4; ++i) {
unsigned seconds_this_century = s[indices[i]];
if (seconds < seconds_this_century)
return i;
seconds -= seconds_this_century;
}
assert(false && "Unreachable");
return -1;
}
// Given a 4 year period where the |leap_index|'th year is a leap year,
// return which of the 4 years this second counter falls into. To
// indicate that none of the 4 years is a leap year (e.g. [1900,1904))
// pass -1 for |leap_index|
static bool which_year_in_4(unsigned &seconds, unsigned &year, int leap_index) {
unsigned s[] = {seconds_per_nl_year, seconds_per_nl_year,
seconds_per_nl_year, seconds_per_nl_year};
if (leap_index >= 0)
s[leap_index] = seconds_per_l_year;
for (unsigned i=0; i < 4; ++i) {
if (seconds < s[i]) {
year = i;
return true;
}
seconds -= s[i];
}
return false;
}
static unsigned which_year_in_century(unsigned &seconds, unsigned century) {
assert(century % 100 == 0 && "Invalid century!");
bool leap = is_leap_year(century);
assert(seconds < seconds_per_l_century && "Invalid seconds!");
unsigned result = 0;
if (!leap) {
assert(seconds < seconds_per_nl_century && "Invalid seconds!");
}
// The first year may or may not be a leap year. So treat the group
// of first 4 years separately, and early-out if the period falls in that
// range.
int leap_idx = (leap) ? 0 : -1;
if (which_year_in_4(seconds, result, leap_idx))
return result;
// Now there are 96 years left, always starting with a leap year. So
// each period has equal length. Find out which one we're in.
unsigned period = seconds / seconds_per_l_4_years;
seconds -= period * seconds_per_l_4_years;
bool success = which_year_in_4(seconds, result, 0);
assert(success);
// Now |result| contains the index of the year, and |seconds| contains
// the remainder (i.e. the number of seconds into the year).
return result;
}
public:
// Determines if year is a leap year
static bool is_leap_year(unsigned year) {
if (year % 4 != 0)
return false;
if (year % 100 != 0)
return true;
if (year % 400 == 0)
return true;
return false;
}
// Finds the first leap year in the range [begin, infinity)
static unsigned first_leap_year(unsigned begin) {
// Iterate from 0 to 8 instead of 0 to 4 since every
// 4 years is not necessarily a leap year (due to centennials)
for (unsigned i=0; i < 8; ++i) {
if (is_leap_year(begin + i))
return begin + i;
}
assert(false && "Unreachable");
}
// Finds the last leap year in the range [0, end]
static unsigned last_leap_year(unsigned end) {
for (unsigned i=0; i < 8; ++i) {
if (is_leap_year(end - i))
return end - i;
}
assert(false && "Unreachable");
}
// Calculate the number of years in the range [begin, end) that are
// leap years.
static unsigned leaps_between(unsigned begin, unsigned end) {
if (end == 0)
return 0;
unsigned f = first_leap_year(begin);
// Note that we are specifically excluding end from the range here,
// so that we can use it in situations where "end" is the /current/
// year, and we haven't yet decided if we're past Feb 29th or not yet
unsigned e = last_leap_year(end - 1);
if (f > e)
return 0;
unsigned distance = e - f;
assert(distance % 4 == 0 && "Unexpected distance");
return distance / 4;
}
unsigned day_of_year() const {
unsigned dy = 0;
for (unsigned i=0; i < month; ++i)
dy += days_per_month[i];
dy += day;
if (!is_leap_year())
return dy;
if ((month == 1 && day == 29) || month > 1)
return dy + 1;
return dy;
}
bool is_leap_year() const {
return is_leap_year(year);
}
unsigned serial() const {
unsigned num_leaps = leaps_between(1900, year);
unsigned years = year - 1900;
unsigned seconds_until_this_year = years * seconds_per_nl_year;
seconds_until_this_year += num_leaps * seconds_per_day;
return seconds_until_this_year + day_of_year() * seconds_per_day;
}
static Date from_serial(unsigned serial) {
unsigned period = serial / seconds_per_centennial_period;
unsigned remainder = serial % seconds_per_centennial_period;
// Now that we know the period, which century within the period
// are we in? The first, second, third, or fourth?
unsigned c = which_century_in_period(remainder);
assert(c < 4 && "Invalid century!");
unsigned century = 1900 + period * 400 + c * 100;
unsigned year = which_year_in_century(remainder, century);
assert(year < 100 && "Invalid year!");
year += century;
unsigned month, day;
which_month_and_day(remainder, year, month, day);
return Date(year, month, day);
}
};
Edit: Ok fixed it up. Again, I haven't even compiled this, and this seemed way more complicated than I expected it to be, so maybe there's an easier solution.
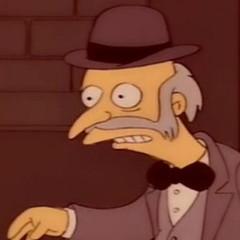
Apologies if this link was given before, but I've found this site pretty useful. I'm a SQL guy trying to learn C# in some down time (yeah, right). There's some good katas here that have helped me grasp some basics and syntax along the way. Still very much a newb at it though. https://www.codewars.com
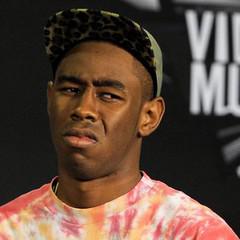
Question:
I'm supposed to be handling security testing for a web app right now (mostly grey box automation but I can go beyond that), and I got absolutely no experience. Like, I don't even know what the baseline security tests are besides single sign on related stuff, and SQL encryption.
Help? Namely, I'm supposed to be working on URL Spoofing tests.
I'm supposed to be handling security testing for a web app right now (mostly grey box automation but I can go beyond that), and I got absolutely no experience. Like, I don't even know what the baseline security tests are besides single sign on related stuff, and SQL encryption.
Help? Namely, I'm supposed to be working on URL Spoofing tests.
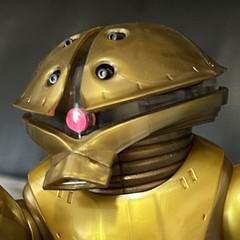
By Double 0 Go To PostQuestion:You may also want to look into cross site scripting and also sql injection (not sure if that's what you meant by sql encryption).
I'm supposed to be handling security testing for a web app right now (mostly grey box automation but I can go beyond that), and I got absolutely no experience. Like, I don't even know what the baseline security tests are besides single sign on related stuff, and SQL encryption.
Help? Namely, I'm supposed to be working on URL Spoofing tests.
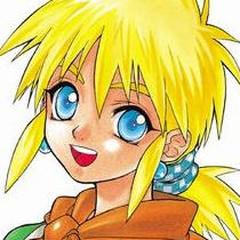
So i have to questions that i need help on. The first one is how can i find the average of even numbers. The second question is how can i find the first number read from the input. Imma leave my code here. edit: its c++ btw
#include <iostream>
#include <iomanip>
using namespace std;
int main()
{
int number,
num1,
zero = 0,
count = 0,
Max_number = 10,
odd = 0,
even = 0;
double eventotal;
cout << "Please enter 10 positive integers seperated by space:\n";
while (count < Max_number)
{
cin >> number;
count++;
if (number == 0)
{
zero++;
}
if (number % 2 == 1)
{
odd += number;
}
if (number % 2 == 0)
{
even += number;
}
}
cout << "Number of zeros " << zero << endl;
cout << "Sum of odd numbers " << odd << endl;
cout << "Average of even numbers: " << eventotal << endl;
cout << "First number read: ";
cout << "\nLast number read: " << number << endl;
return 0;
}
#include <iostream>
#include <iomanip>
using namespace std;
int main()
{
int number,
num1,
zero = 0,
count = 0,
Max_number = 10,
odd = 0,
even = 0;
double eventotal;
cout << "Please enter 10 positive integers seperated by space:\n";
while (count < Max_number)
{
cin >> number;
count++;
if (number == 0)
{
zero++;
}
if (number % 2 == 1)
{
odd += number;
}
if (number % 2 == 0)
{
even += number;
}
}
cout << "Number of zeros " << zero << endl;
cout << "Sum of odd numbers " << odd << endl;
cout << "Average of even numbers: " << eventotal << endl;
cout << "First number read: ";
cout << "\nLast number read: " << number << endl;
return 0;
}
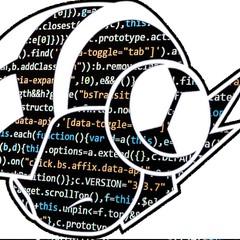
So, I know Java, JSF, HTML, and c++ but would like to learn some more languages that my school doesn't offer like Python and such. Just jump in online or what resources do you guys recommend?
Also interested in game design, I have game maker pro and have made some silly games in SFML for C++ but am more interested in VR development. What suggestions do you all have? ALSO interactive UX/UI design courses since my school doesn't offer any at all.
Hey man, I can help you with this later but a suggestion, it would be a lot easier if you post the code in the ["code"] [/"code"] brackets, (remove the quotes) or link to another site like pastebin which will maintain your formatting.
Also interested in game design, I have game maker pro and have made some silly games in SFML for C++ but am more interested in VR development. What suggestions do you all have? ALSO interactive UX/UI design courses since my school doesn't offer any at all.
By Holtlegna Go To Postcode
Hey man, I can help you with this later but a suggestion, it would be a lot easier if you post the code in the ["code"] [/"code"] brackets, (remove the quotes) or link to another site like pastebin which will maintain your formatting.
int main() {
std::cout << "See how easier this is to read?" << std::endl;
}
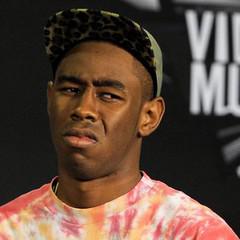
By Kibner Go To PostYou may also want to look into cross site scripting and also sql injection (not sure if that's what you meant by sql encryption).
Thanks for the link! Don't worry about SQL Injection/encryption. I got that already.
For me, I'm more worried about just general security tests and URL Spoofing. Gonna try that cross site scripting though.
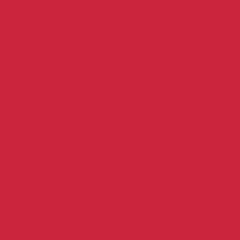
By Holtlegna Go To PostSo i have to questions that i need help on. The first one is how can i find the average of even numbers. The second question is how can i find the first number read from the input. Imma leave my code here.
First, read all the numbers:
cout << "Please enter 10 positive integers seperated by space:\n";
unsigned numbers[10];
for (int i=0; i < 10; ++i)
cin >> numbers[i];
Now if you want to find the first number read from the input, it's just whatever is in numbers[0].
unsigned first_number = numbers[0];
So how do you find the average of numbers? You take the sum, then divide by the count. So if you want to find the average of even numbers, you need to find the sum of even numbers and the count of even numbers. This is a straightforward modification to the original program.
cout << "Please enter 10 positive integers seperated by space:\n";
unsigned numbers[10];
unsigned sum_of_evens = 0;
unsigned count_of_evens = 0;
for (int i=0; i < 10; ++i) {
cin >> numbers[i];
if (numbers[i] % 2 == 0) {
sum_of_evens += numbers[i];
++count_of_evens;
}
}
Now you only need 1 more step to find the average of even numbers.
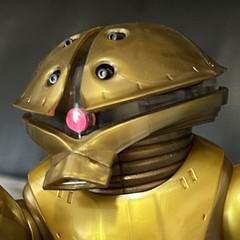
By TI92 Go To PostSo, I know Java, JSF, HTML, and c++ but would like to learn some more languages that my school doesn't offer like Python and such. Just jump in online or what resources do you guys recommend?Pick a project that seems interesting to you (like a beer rater or something, I dunno) and code it in whatever language(s) you want to learn. Use online resources to learn how to do specific tasks in that language if you are struggling. Learn design patterns and google for best practices, as well.
Also interested in game design, I have game maker pro and have made some silly games in SFML for C++ but am more interested in VR development. What suggestions do you all have? ALSO interactive UX/UI design courses since my school doesn't offer any at all.
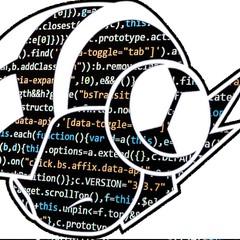
By Kibner Go To PostPick a project that seems interesting to you (like a beer rater or something, I dunno) and code it in whatever language(s) you want to learn. Use online resources to learn how to do specific tasks in that language if you are struggling. Learn design patterns and google for best practices, as well.
I guess I was more wondering if there were good courses on design because not all of it is intuitive. I love material design from google but Matias Duarte was an art person as well as a technical person so I feel there must be some concepts to learn there, no?
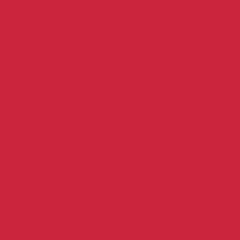
Hi everyone,
Nice to see a healthy mix of software people here. I'm a recovering software engineer who recently moved into UX design. But I was a jack of all trades who specialized in .Net, C++, iOS, Android, node, and client side JavaScript. My last major project before switching was developing a cloud based real time device monitoring system for a safety critical environment, leading the android development, the node webserver, and the angular client. Still have that programmer's itch though, to the point that I find myself finding any excuse I can to write some code. My main interests at this point (besides for UX stuff) is node and vue.js and would be glad to lend my expertise to help out.
If you like material design, definitely start of with giving a good read of the material design spec, https://material.io, and paying attention to the little details and how they repeat for different controls. Design is largely about consistency and making interactions the same for similar actions, so make sure to realize what the patterns are and how they repeat in different controls. For learning more about design and usability, I'd suggest reading some articles from the Nielson Norman Group, https://www.nngroup.com/articles/, and browsing around medium. Design is definitely art but also about understanding your user and simplifying their interactions.
Nice to see a healthy mix of software people here. I'm a recovering software engineer who recently moved into UX design. But I was a jack of all trades who specialized in .Net, C++, iOS, Android, node, and client side JavaScript. My last major project before switching was developing a cloud based real time device monitoring system for a safety critical environment, leading the android development, the node webserver, and the angular client. Still have that programmer's itch though, to the point that I find myself finding any excuse I can to write some code. My main interests at this point (besides for UX stuff) is node and vue.js and would be glad to lend my expertise to help out.
By TI92 Go To PostI guess I was more wondering if there were good courses on design because not all of it is intuitive. I love material design from google but Matias Duarte was an art person as well as a technical person so I feel there must be some concepts to learn there, no?
If you like material design, definitely start of with giving a good read of the material design spec, https://material.io, and paying attention to the little details and how they repeat for different controls. Design is largely about consistency and making interactions the same for similar actions, so make sure to realize what the patterns are and how they repeat in different controls. For learning more about design and usability, I'd suggest reading some articles from the Nielson Norman Group, https://www.nngroup.com/articles/, and browsing around medium. Design is definitely art but also about understanding your user and simplifying their interactions.
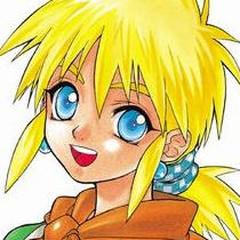
By c++ Go To PostFirst, read all the numbers:Thank you :Dcout << "Please enter 10 positive integers seperated by space:\n"; unsigned numbers[10]; for (int i=0; i < 10; ++i) cin >> numbers[i];
Now if you want to find the first number read from the input, it's just whatever is in numbers[0].unsigned first_number = numbers[0];
So how do you find the average of numbers? You take the sum, then divide by the count. So if you want to find the average of even numbers, you need to find the sum of even numbers and the count of even numbers. This is a straightforward modification to the original program.cout << "Please enter 10 positive integers seperated by space:\n"; unsigned numbers[10]; unsigned sum_of_evens = 0; unsigned count_of_evens = 0; for (int i=0; i < 10; ++i) { cin >> numbers[i]; if (numbers[i] % 2 == 0) { sum_of_evens += numbers[i]; ++count_of_evens; } }
Now you only need 1 more step to find the average of even numbers.
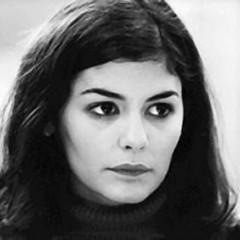
By TI92 Go To PostSo, I know Java, JSF, HTML, and c++ but would like to learn some more languages that my school doesn't offer like Python and such. Just jump in online or what resources do you guys recommend?Python is great and easy to learn. It is big in the data analytics/machine learning/big data field right now, which is kind of a big thing with social media and databases. It was actually the first thing i got into on my own years ago and have been getting into it again now that im back in school. Also started with VB. Both are easy to pick up. Python is very intuitive, at least to me. Udemy and lynda.com have a bunch of useful vid tutorials, but honestly even some ebook pdf is good for python.
Also interested in game design, I have game maker pro and have made some silly games in SFML for C++ but am more interested in VR development. What suggestions do you all have? ALSO interactive UX/UI design courses since my school doesn't offer any at all.
Hey man, I can help you with this later but a suggestion, it would be a lot easier if you post the code in the ["code"] [/"code"] brackets, (remove the quotes) or link to another site like pastebin which will maintain your formatting.int main() { std::cout << "See how easier this is to read?" << std::endl; }
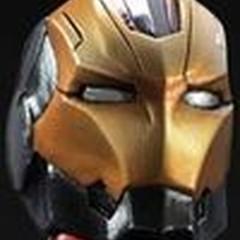
By Smokey Go To PostAlright so programming gurus , recommend me a good language that I can use to get back I to programming. I mentioned that I had to take some classes in college, so the basic concepts are still with me (I think). Has Nelo posted in here? I know he was in the self teach grind for a long time.I learned Ruby and Python at the same time, but for more DevOps stuff. I will say that I prefer Python when I want to throw up something quick, but Ruby/RoR when I want to do something complex.
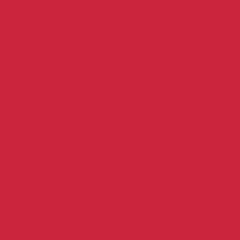
By TI92 Go To PostAlso interested in game design, I have game maker pro and have made some silly games in SFML for C++ but am more interested in VR development. What suggestions do you all have? ALSO interactive UX/UI design courses since my school doesn't offer any at all.
I took Ben tristens udemy course on unreal and thought it was great! I couldn't finish because my laptop couldn't keep up but when I build a new desktop I plan to take all of his courses.
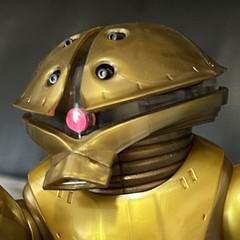
I was just linked this tool and it is really cool: http://cosette.cs.washington.edu
It determines if two SQL statements are equivalent or not.
e: if the statements are not equivalent, it will return a counter-example
It determines if two SQL statements are equivalent or not.
e: if the statements are not equivalent, it will return a counter-example
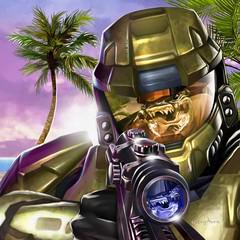
By Randolph Freelander Go To PostI'd offer to pitch in, but this stack is almost completely foreign. 99.98% of my work is in .NET.
You and me both, I used to custom code all day everyday in a couple of languages but now I mostly just project manage .NET C# projects and solution design, test etc. After years of not directly coding from the ground up and the rise of CMS/libraries I just lost the will, practice or need to go from 0 to 100 coding wise. I'd be more dangerous than useful within writing code, but the old problem solving and logical thinking remained...at least I think it did.
React however is a nice framework for this site to be running on. The server side of nginx interests me, obviously the performance on this site is holding up to the GAF spillover very well so far. Nice work there guys'n'gals. I've moved from dedicated windows servers to Azure cloud hosting and now are pushing back to differing platforms for eCommerce solutions e.g. Linux, nginx, php over .NET etc.
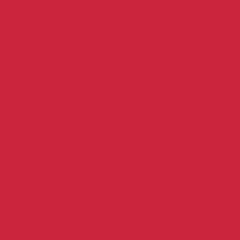
Ok because I just couldn't resist, I did the whole date exercise using modern C++.
I really like the fact that static_assert + constexpr allows you to basically write tests at compile time, don't even have to compile your program to see if it works or not, because the IDE just gives you squigglies immediately after you type something if it fails.
This allowed me to fix a few bugs in my original implementation, and now I know it's correct even though I *still* haven't compiled or run it.
I only did this for the core routines though, so it's possible a bug crept into the class itself. But the class is a really simple layer on top of all the constexpr functions, so it should be fairly straightforward to just go add some test cases after each routine using static_assert(). Then you can just tell your prof that he doesn't even need to waste time running it, because it compiled.
(And for the record, I went out of my way to use all this modern stuff specifically so that you can't copy my code. You should still understand it and re-write it yourself ;-)
#include <assert.h>
#include <optional>
#include <tuple>
#include <utility>
constexpr unsigned spd = 24 * 60 * 60;
// Returns true if the given year is a leap year, false otherwise. Accounts for
// leap centuries.
constexpr bool is_leap_year(unsigned year) {
if (year % 4 != 0)
return false;
if (year % 100 != 0)
return true;
if (year % 400 == 0)
return true;
return false;
}
static_assert(is_leap_year(2000));
static_assert(is_leap_year(1904));
static_assert(!is_leap_year(1900));
constexpr unsigned dpm(unsigned year, unsigned month) {
assert(month < 12 && "Invalid month!");
constexpr unsigned dpm_init[] = {
31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31
};
unsigned days = dpm_init[month];
if (month == 1 && is_leap_year(year))
++days;
return days;
}
// Number of seconds in the given year, automatically determining if the year is a
// leap year.
constexpr unsigned spy(unsigned year) {
return 365 * spd + (is_leap_year(year) ? spd : 0);
}
// Number of seconds in a given century, accounting for the fact that the
// century may or may not be a leap century.
constexpr unsigned leaps_per_century(unsigned century) {
assert(century % 100 == 0);
return (century % 400 == 0) ? 25 : 24;
}
static_assert(leaps_per_century(1900) == 24);
static_assert(leaps_per_century(2000) == 25);
// Number of seconds in a given century, accounting for the fact that the
// century may or may not be a leap century.
constexpr unsigned spc(unsigned century) {
assert(century % 100 == 0);
unsigned l = leaps_per_century(century);
unsigned nl = 100 - l;
return l * spy(true) + nl * spy(false);
}
// Returns the number of seconds in a period of 4-consecutive centuries.
constexpr unsigned sp400(unsigned century) {
assert(century % 100 == 0);
return spc(century) + spc(century+100) + spc(century+200) + spc(century+300);
}
static_assert(sp400(1900) == sp400(2000));
static_assert(sp400(1900) == sp400(2000));
// Finds the first leap year in the range [begin, infinity)
constexpr unsigned first_leap(unsigned begin) {
// Iterate from 0 to 8 instead of 0 to 4 since every
// 4 years is not necessarily a leap year (due to centennials)
for (unsigned i = 0; i < 8; ++i) {
if (is_leap_year(begin + i))
return begin + i;
}
assert(false && "Unreachable");
}
static_assert(first_leap(1896) == 1896);
static_assert(first_leap(1897) == 1904);
static_assert(first_leap(1898) == 1904);
static_assert(first_leap(1899) == 1904);
static_assert(first_leap(1900) == 1904);
static_assert(first_leap(1901) == 1904);
static_assert(first_leap(1902) == 1904);
static_assert(first_leap(1903) == 1904);
static_assert(first_leap(1904) == 1904);
static_assert(first_leap(1905) == 1908);
static_assert(first_leap(2000) == 2000);
// Finds the last leap year in the range [0, end]
constexpr unsigned last_leap(unsigned end) {
for (unsigned i = 0; i < 8; ++i) {
if (is_leap_year(end - i))
return end - i;
}
assert(false && "Unreachable");
}
static_assert(last_leap(1896) == 1896);
static_assert(last_leap(1897) == 1896);
static_assert(last_leap(1898) == 1896);
static_assert(last_leap(1899) == 1896);
static_assert(last_leap(1900) == 1896);
static_assert(last_leap(1901) == 1896);
static_assert(last_leap(1902) == 1896);
static_assert(last_leap(1903) == 1896);
static_assert(last_leap(1904) == 1904);
static_assert(last_leap(1905) == 1904);
static_assert(last_leap(2000) == 2000);
// Calculate the number of years in the range [begin, end) that are
// leap years. Note that we exclude the end year in this case.
constexpr unsigned leaps_between(unsigned begin, unsigned end) {
if (end == 0)
return 0;
unsigned f = first_leap(begin);
// Note that we are specifically excluding end from the range here,
// so that we can use it in situations where "end" is the /current/
// year, and we haven't yet decided if we're past Feb 29th or not yet
unsigned e = last_leap(end - 1);
if (f > e)
return 0;
unsigned distance = e - f;
assert(distance % 4 == 0 && "Unexpected distance");
return distance / 4 + 1;
}
static_assert(leaps_between(1900, 2000) == leaps_per_century(1900));
static_assert(leaps_between(2000, 2100) == leaps_per_century(2000));
// Number of seconds in a 4 year period starting with |year|
constexpr unsigned sp4(unsigned year) {
bool has_leap = leaps_between(year, year + 4) > 0;
return 4 * spy(false) + (has_leap ? spd : 0);
}
// Given a number of seconds from Jan 1 for a particular year, find the
// corresponding month and day in that year.
constexpr std::pair<unsigned, unsigned=""> month_and_day(unsigned seconds, unsigned year) {
assert(seconds < spy(year) && "seconds out of bound for the given year!");
bool leap = is_leap_year(year);
// Iterate the months of the specified year, successively subtracting out the
// number of seconds from that month. Eventually the number of seconds
// "remaining" in the year should be less than the number of seconds in the
// current month, which is the indication that we've found the month.
for (unsigned i = 0; i < 12; ++i) {
unsigned seconds_this_month = dpm(i, year) * spd;
if (seconds < seconds_this_month)
return std::make_pair(i, seconds / spd);
seconds -= seconds_this_month;
}
assert(false && "Invalid seconds count!");
}
// Given the number of seconds from the start of a 4 century period beginning at
// |century|, which century are we in? On output, returns the index of the
// century [0,4) we're in within this period, as well as the number of seconds
// "into" the given century.
constexpr std::pair<unsigned, unsigned=""> which_century_in_period(unsigned seconds, unsigned century) {
assert(seconds < sp400(0));
// Initialize this array with the second counts of 2 centuries. One that is
// a known leap-century, and one which is a known non-leap century.
constexpr unsigned s[] = { spc(1900), spc(2000) };
unsigned indices[] = {
is_leap_year(century),
is_leap_year(century+100),
is_leap_year(century+200),
is_leap_year(century+300)
};
for (unsigned i = 0; i < 4; ++i) {
unsigned seconds_this_century = s[indices[i]];
if (seconds < seconds_this_century)
return std::make_pair(i, seconds);
seconds -= seconds_this_century;
}
assert(false && "Unreachable");
return std::make_pair(0,0);
}
// Given a 4 year period where the |leap_index|'th year is a leap year, return
// a pair whose first member is the index [0,4) of the year year this second
// counter falls into, and whose second value is the number of seconds into
// that year.
constexpr std::optional<std::pair<unsigned, unsigned="">>
which_year_in_4(unsigned seconds, unsigned year) {
unsigned s[] = {
spy(year), spy(year + 1), spy(year + 2), spy(year + 3)
};
for (unsigned i = 0; i < 4; ++i) {
if (seconds < s[i])
return std::make_pair(i, seconds);
seconds -= s[i];
}
return {};
}
constexpr std::pair<unsigned, unsigned="">
which_year_in_century(unsigned seconds, unsigned century) {
assert(century % 100 == 0 && "Invalid century!");
assert(seconds < spc(century) && "Invalid seconds!");
// The first year may or may not be a leap year. So treat the group
// of first 4 years separately, and early-out if the period falls in that
// range.
auto result = which_year_in_4(seconds, century);
if (result)
return *result;
// Now there are 96 years left, always starting with a leap year. So each
// period of 4 years is identical. So we can just divide to find out which
// 4 year cycle we're in.
seconds -= sp4(century);
unsigned periods = seconds / sp4(century + 4);
seconds -= periods * sp4(century + 4);
// Now we're down to only 4 years remaining, starting with a leap year.
// |seconds| must lie in one of these 4 years.
result = which_year_in_4(seconds, century + (periods + 1) * 4);
assert(result.has_value());
// Now |result| contains the index of the year, and |seconds| contains
// the remainder (i.e. the number of seconds into the year).
return *result;
}
class Date {
private:
unsigned year; // assert(year >= 1900)
unsigned month; // month in [0,12)
unsigned day; // day in [0,31)
public:
Date(unsigned m, unsigned d, unsigned y)
: month(m), day(d), year(y) {}
unsigned day_of_year() const {
unsigned dy = 0;
for (unsigned i = 0; i < month; ++i)
dy += dpm(year, i);
return dy + day;
}
bool is_leap_year() const {
return ::is_leap_year(year);
}
unsigned serial() const {
// Number of leap years from [1900,year). Note that we exclude this year,
// because we may still be <= Feb 28th, in case we shouldn't factor in the
// leap day.
unsigned l = leaps_between(1900, year);
unsigned nl = (year - 1900) - l;
// Use 2000 and 2001 to get seconds for leap and non-leap years,
// respectively. Since they're constexpr this incurs no additional
// cost.
unsigned serial_until_this_year = l * spy(2000) + nl * spy(2001);
return serial_until_this_year + day_of_year() * spd;
}
static Date from_serial(unsigned serial) {
// Here we just need the seconds for an arbitrary 400 year centennial
// period. Since all values are the same, just use sp400(0).
unsigned period = serial / sp400(0);
unsigned remainder = serial % sp400(0);
unsigned periody = 1900 + period * 400;
// Now that we know the period, which century within the period are we
// in? The first, second, third, or fourth?
unsigned ci = 0, yi = 0;
std::tie(ci, remainder) = which_century_in_period(remainder, periody);
assert(ci < 4 && "Invalid century!");
unsigned century = periody + ci * 100;
std::tie(yi, remainder) = which_year_in_century(remainder, century);
assert(yi < 100 && "Invalid year!");
unsigned year = century + yi;
unsigned month, day;
std::tie(month, day) = month_and_day(remainder, year);
return Date(year, month, day);
}
};
int main()
{
return 0;
}
I really like the fact that static_assert + constexpr allows you to basically write tests at compile time, don't even have to compile your program to see if it works or not, because the IDE just gives you squigglies immediately after you type something if it fails.
This allowed me to fix a few bugs in my original implementation, and now I know it's correct even though I *still* haven't compiled or run it.
I only did this for the core routines though, so it's possible a bug crept into the class itself. But the class is a really simple layer on top of all the constexpr functions, so it should be fairly straightforward to just go add some test cases after each routine using static_assert(). Then you can just tell your prof that he doesn't even need to waste time running it, because it compiled.
(And for the record, I went out of my way to use all this modern stuff specifically so that you can't copy my code. You should still understand it and re-write it yourself ;-)
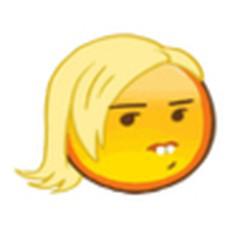
By Script Kiddies LLC Go To PostI learned Ruby and Python at the same time, but for more DevOps stuff. I will say that I prefer Python when I want to throw up something quick, but Ruby/RoR when I want to do something complex.
I do devops, but I went Perl -> Python -> Ruby. I still do some Python here and there but most of what I do is Ruby since we use Chef pretty extensively at work. If I want to whip something together fast I've always gone with good old bash.
Got a bit into Go recently because I absolutely love the idea of a Docker image with just a single statically compiled binary. Traefik inspired me to tinker with that when I tried to execute a shell in a running container and realized there was none.
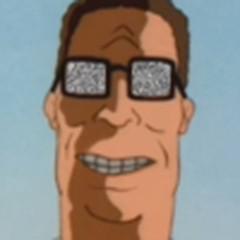
By jezze Go To PostWhen this goes open source, I'm down to contribute. I'll brush up on my python/Django and PostgreSQL until then :).DjangreSQL bros. Trying to come up with time to make my Angular 2 app with a Django/Postgre API but shit I got too many things to be studying and learning right now if I want to actually get a job in WebDev. Started talking more intently to recruiters though, fingers crossed.
Anyone have experience with Leaflet.js and dynamic loading of map points?
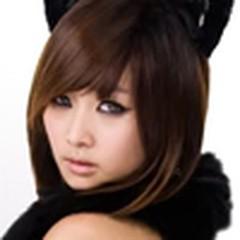
By Frankenstrat Go To PostDjangreSQL bros. Trying to come up with time to make my Angular 2 app with a Django/Postgre API but shit I got too many things to be studying and learning right now if I want to actually get a job in WebDev. Started talking more intently to recruiters though, fingers crossed.Dynamic maps on native android, yes. Leaflet, no.
Anyone have experience with Leaflet.js and dynamic loading of map points?
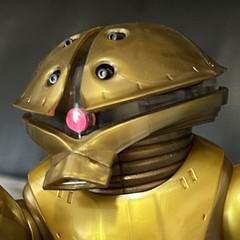
Man, fuck a Joomla. I have only had to work with it twice, but it has been such a pain in the ass. I mean, I know more about it now, but yeah. It's just such a pain to do custom stuff from the administrator side.
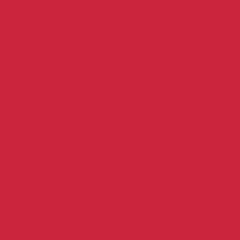
Hey guys, I was wondering if anyone could point me in the direction of some good Spring/Spring Boot tutorials/videos/books etc..
I'm already familiar with the very basics of it as I've built one or two basic applications with it but I'm looking to get more in depth knowledge/explanations on it. Whenever I check on for example Youtube I can't seem to find any good materials on it which is weird as I can find videos on almost anything else development related.
Any help would be appreciated, thanks!
I'm already familiar with the very basics of it as I've built one or two basic applications with it but I'm looking to get more in depth knowledge/explanations on it. Whenever I check on for example Youtube I can't seem to find any good materials on it which is weird as I can find videos on almost anything else development related.
Any help would be appreciated, thanks!
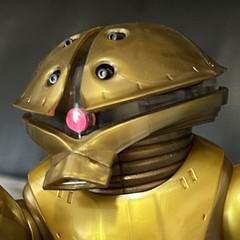
By Ryujin Go To PostHey guys, I was wondering if anyone could point me in the direction of some good Spring/Spring Boot tutorials/videos/books etc..
I'm already familiar with the very basics of it as I've built one or two basic applications with it but I'm looking to get more in depth knowledge/explanations on it. Whenever I check on for example Youtube I can't seem to find any good materials on it which is weird as I can find videos on almost anything else development related.
Any help would be appreciated, thanks!
Try looking at a few of these sites:
https://www.tutorialspoint.com/spring/
https://courses.caveofprogramming.com/p/the-java-spring-tutorial
https://www.udemy.com/courses/search/?q=spring&src=ukw
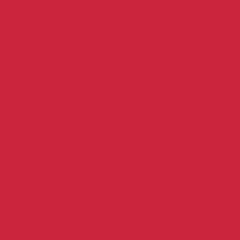
By Kibner Go To PostTry looking at a few of these sites:
https://www.tutorialspoint.com/spring/
https://courses.caveofprogramming.com/p/the-java-spring-tutorial
https://www.udemy.com/courses/search/?q=spring&src=ukw
Thanks! I'll check those out and see how I progress!
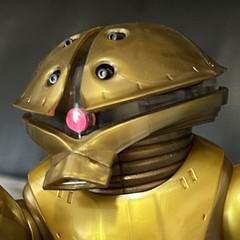
By Ryujin Go To PostThanks! I'll check those out and see how I progress!I haven't used any of those sites before, so be sure to do your due diligence before forking over any money for a course.
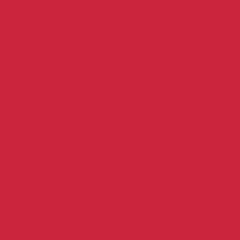
By Kibner Go To PostI haven't used any of those sites before, so be sure to do your due diligence before forking over any money for a course.
Ah no worries I've used Udemy before they can be hit or miss but the course I picked seems focused and was only 12 euro so not too bad. I can always get a refund if I'm not happy with it.
I didn't bother with the caveofprogramming one once I saw it was Spring 4 and a paid course, too unknown of a source.
TutorialsPoint can be a good free source of info, only problem is they tend to be a little out of date. But there will likely be some useful info there.
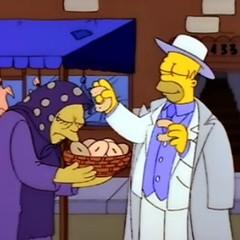
Hey gang,
I don't know if this is the right thread for this, but I assume you're all boffins with tech.
So yours truly just purchased a Sony Experia XZ Premium (My first phone since 2013), very sweet piece of kit.
I have just completed transferring all my files over from my old phone. However, when I attempted to switch out the old micro sd card to the new phone, an alert would come up saying its corrupted and I should tap to fix it by formatting the card, the sd card refuses to fully format and does not appear at all.
Any attempt to format would reach 20% and stop. Every attempt at formatting has failed, I don't have a card reader to attach it directly to the pc. I tried formatting it on my sister's phone but it doesn't work, she uses a Huawei and it only suggests erasing it not formatting it.
Any tips or tricks you can provide would be greatly appreciated.
I don't know if this is the right thread for this, but I assume you're all boffins with tech.
So yours truly just purchased a Sony Experia XZ Premium (My first phone since 2013), very sweet piece of kit.
I have just completed transferring all my files over from my old phone. However, when I attempted to switch out the old micro sd card to the new phone, an alert would come up saying its corrupted and I should tap to fix it by formatting the card, the sd card refuses to fully format and does not appear at all.
Any attempt to format would reach 20% and stop. Every attempt at formatting has failed, I don't have a card reader to attach it directly to the pc. I tried formatting it on my sister's phone but it doesn't work, she uses a Huawei and it only suggests erasing it not formatting it.
Any tips or tricks you can provide would be greatly appreciated.
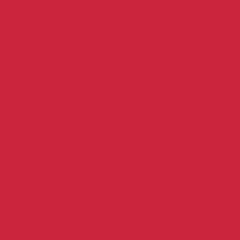
Sounds like the SD card is corrupted. Also to the best of my knowledge if you are formatting the card then that will automatically erase the file contents. It sounds like your initial attempt to format the card failed causing it to corrupt the data/card.
It doesn't look like you have many options sorry.
It doesn't look like you have many options sorry.